Maelstrom CMS Toolkit
A CMS toolkit for intermediate to advanced development teams who love React, Tailwind & Laravel.
Maelstrom is a collection of Laravel and React components to help you flesh out your own control panels using as much of vanilla Laravel as possible.
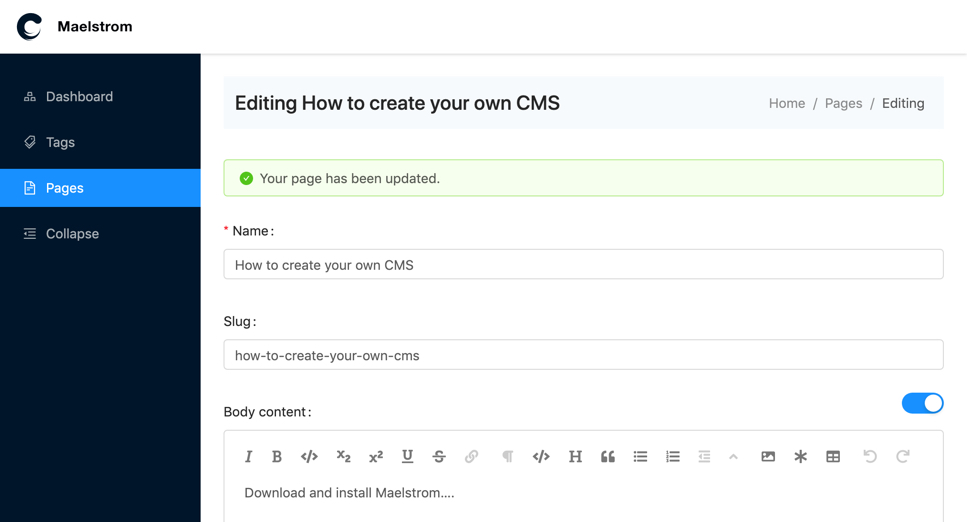
Why use Maelstrom?
Unlike other solutions such as Nova - Maelstrom doesn't impose any ways on doing things, we simply provide you various helpers to either use, or ignore - allowing you to implement things exactly as you need. Utilising the IoC container, waterfall includes, extendable UI components and a controversial god class, everything can be changed to suit your needs with ease.
The Stack
Maelstrom uses a cutting edge stack to afford you the ability to create the most advanced systems, unlike many other systems we don't use any Bootstrap themes, it's a pure utility class based interface using Tailwind and the UI library from Ant Design to allow you to create what ever you need.
Laravel 5.7+
Maelstrom is based on the incredibly developer friendly Laravel, we use as much of vanilla Laravel as possible which means if Laravel allows you to do it, then we allow you to do it. We even use the Mix asset pipeline to allow you the up-most control.
React 16+
We love Vue, but the React eco-system is beautifully rich, so by opting for React you have access to a plethora of extremely durable libraries, including Ant - and by using the class syntax, everything is easily extendable to make your own modifications.
Ant Design
Possible one of the most modern and eye-catching design systems currently available, with a myriad of highly flexible components to build your systems. We use only a handful of their features, however you have access to use them all!
Tailwind
Not forgetting the incredible Tailwind, avoiding the path of themes and going down a utility route, allows you to have the most customised UIs possible, we impose no design restrictions on you, letting you write as much or as little code as you like.